EntryPoint should be updated by adding some values to UserData and passing the user object to ReadView's presenter.
public class EntryPoint
{
[STAThread]
static void Main()
{
MainForm mainForm = new MainForm();
UserData userData = createUser();
new MainPresenter(mainForm, userData, createViewFactory(userData));
Application.Run(mainForm);
}
private static UserData createUser()
{
UserData user = new UserData();
user.Name = "John Doe";
user.JobTitle = "Rockstar";
user.PhoneNumber = "212-555-1212";
return user;
}
private static ViewFactory createViewFactory(UserData user)
{
return new ViewFactory(getViews(user));
}
private static UserControl[] getViews(UserData user)
{
return new UserControl[] { getReadView(user), getUpdateView(user) };
}
private static UserControl getReadView(UserData user)
{
ReadView view = new ReadView();
new ReadPresenter(view, user);
return view;
}
private static UserControl getUpdateView(UserData user)
{
return new UpdateView();
}
}
ReadView's presenter handles the logic that puts the correct data in the ReadView.
public class ReadPresenter
{
private readonly ReadView view;
private readonly UserData user;
public ReadPresenter(ReadView view, UserData user)
{
this.view = view;
this.user = user;
push();
}
private void push()
{
view.NameLabel.Text = user.Name;
view.JobLabel.Text = user.JobTitle;
view.PhoneLabel.Text = user.PhoneNumber;
}
}
The only other changes that need to be made are making the NameLabel, JobLabel, and PhoneLabel public on the view.
The result is a dumb view and all the behavior is in the Presenter. The view should look similar to this and be working at this point if you click on "View."
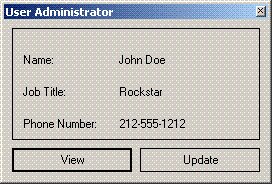
In Part III I'll create the presenter for the UpdateView.
publiv modifier on the label .... i wouldn't do that
ReplyDeleteMaking the Controls public is the easy solution. However, when you are ready for the more robust, testable version you should abstract an interface and provide access to the controls via public properties. An example of this can be seen in the View Observer pattern I previously blogged about.
ReplyDelete